Exploring TypeScript in React: Why It Matters
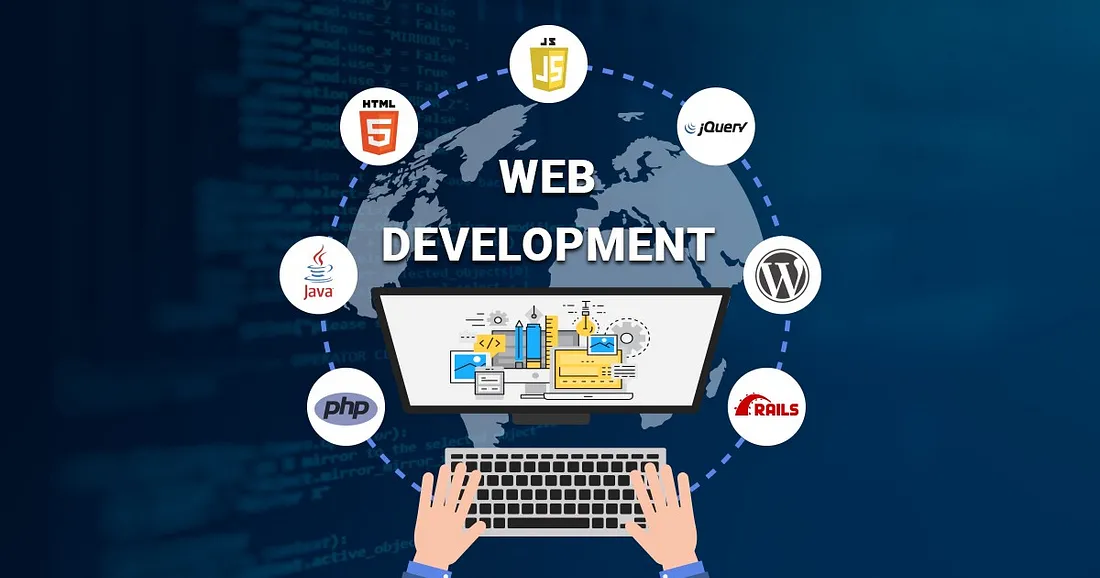
Introduction
As web applications become more complex, maintaining type safety and catching errors early is crucial. TypeScript, a statically-typed superset of JavaScript, offers a solution to this problem by providing strong typing and improved tooling. When used with React, TypeScript can enhance the development process by making code more predictable and easier to debug.
The Benefits of TypeScript
TypeScript introduces static types, which help developers catch errors at compile time rather than runtime. This reduces the chances of unexpected bugs and makes code more maintainable. Additionally, TypeScript provides better IntelliSense support, which improves the developer experience in modern editors like VSCode.
Type Safety in React Components
One of the primary benefits of TypeScript is the ability to define the types of props and state in React components. This ensures that your components are used correctly throughout your application, reducing the risk of bugs.
Example: Typing Props in a React Component
Here's how you can use TypeScript to define types for props in a React component:
interface ButtonProps {
label: string;
onClick: () => void;
}
const Button: React.FC<ButtonProps> = ({ label, onClick }) => {
return <button onClick={onClick}>{label}</button>;
};
Working with TypeScript and Redux
TypeScript also plays well with Redux, allowing you to define the shape of your state and actions. This leads to more predictable state management and better code organization.
Conclusion
Integrating TypeScript with React brings numerous benefits, from catching errors early to improving the developer experience. By adopting TypeScript, you can build more robust, scalable applications that are easier to maintain and extend.