Optimizing React Performance: Best Practices
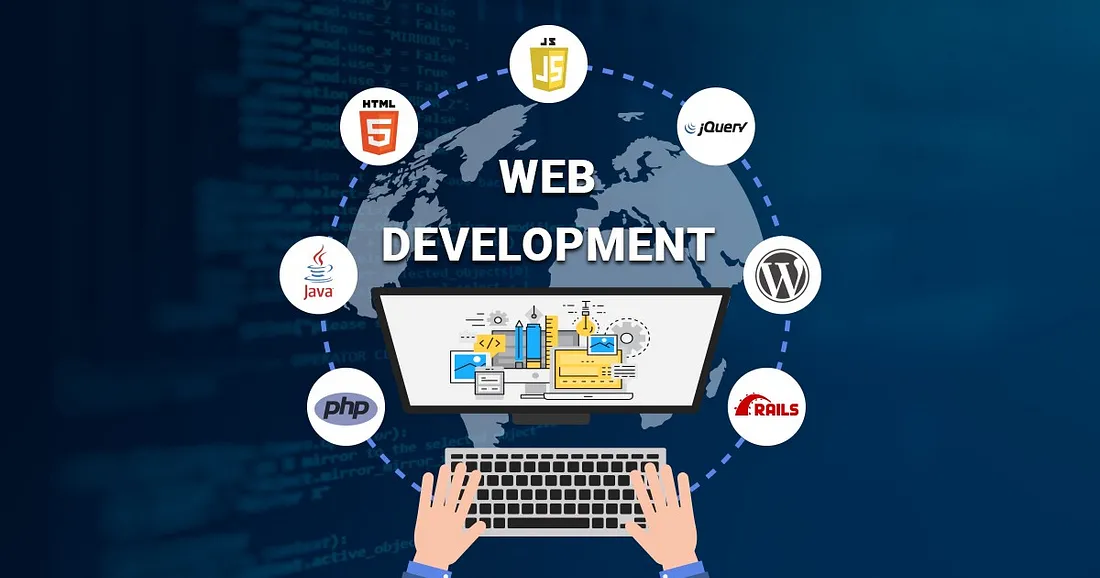
Introduction
As React applications grow in complexity, ensuring optimal performance becomes increasingly important. Slow rendering, unnecessary re-renders, and large bundle sizes can negatively impact the user experience. In this blog, we'll explore some best practices for optimizing React performance.
Memoization with React.memo
and useMemo
One of the most common causes of performance issues in React is unnecessary re-renders. Memoization helps prevent these by caching the results of expensive calculations or by ensuring that components only re-render when their props change.
React.memo
: This higher-order component memoizes a component, preventing it from re-rendering if its props haven't changed.useMemo
: This hook memoizes a value, recomputing it only when its dependencies change.
Example: Using React.memo
to Optimize a Component
import React from 'react';
const ExpensiveComponent = React.memo(({ data }) => {
// expensive calculations here
return <div>{data}</div>;
});
Code Splitting with React.lazy
Code splitting allows you to load parts of your application on demand, reducing the initial load time. React provides built-in support for code splitting with React.lazy
and Suspense
.
Example: Implementing Code Splitting
import React, { Suspense, lazy } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
export default App;
Avoiding Inline Functions in JSX
Inline functions can cause unnecessary re-renders because they create new function instances on every render. To avoid this, define functions outside of your JSX or use useCallback
to memoize them.
Example: Using useCallback
to Prevent Unnecessary Re-renders
import React, { useCallback } from 'react';
function MyComponent() {
const handleClick = useCallback(() => {
// handle click
}, []);
return <button onClick={handleClick}>Click me</button>;
}
Conclusion
Optimizing React performance requires a combination of best practices and careful consideration of how your components are rendered. By implementing memoization, code splitting, and other performance-enhancing techniques, you can create applications that are fast, responsive, and provide a great user experience.