Understanding React Hooks: A Comprehensive Guide
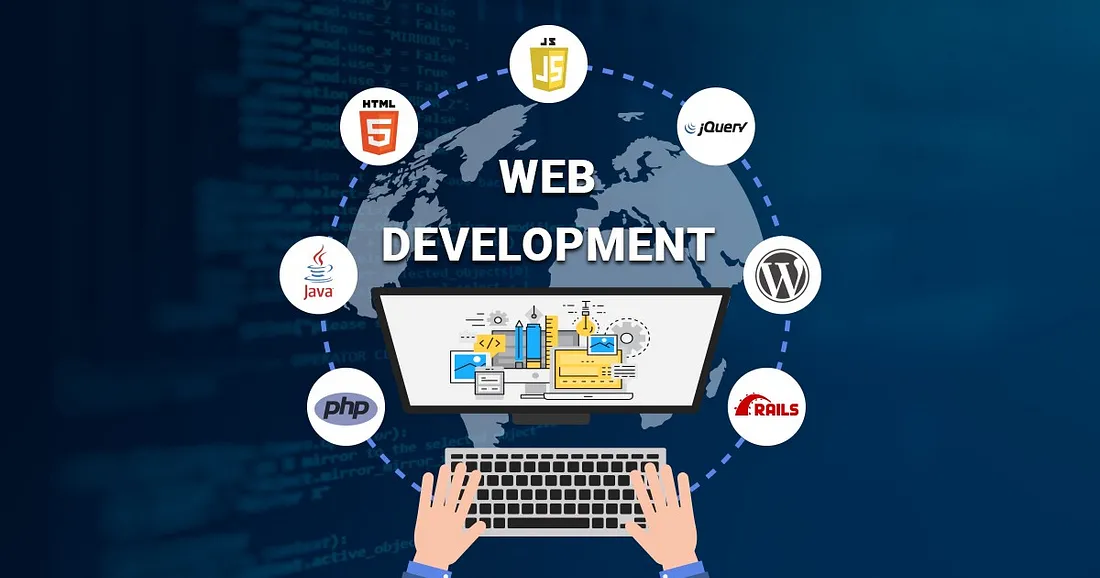
Introduction
React has transformed the way we build user interfaces by embracing a declarative approach. With the introduction of hooks in React 16.8, developers were given powerful tools to manage state and side effects in functional components. This marked a significant shift from class components, enabling more reusable and maintainable code.
What Are Hooks?
Hooks are special functions that allow you to "hook into" React features. For example, useState
lets you add state to functional components, while useEffect
allows you to perform side effects, such as data fetching or subscriptions.
Why Use Hooks?
Before hooks, managing state and side effects in functional components was a challenge, often leading developers to rely on class components. Hooks simplify this process by allowing you to organize logic by behavior rather than lifecycle methods. This makes your code cleaner, more readable, and easier to maintain.
Common Hooks
useState
: Adds local state to functional components.useEffect
: Performs side effects, such as fetching data or subscribing to events.useContext
: Accesses the context object created byReact.createContext
.
Building a Counter with useState
Here's an example of a simple counter using the useState
hook:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Counter;
Conclusion
Hooks have revolutionized the way we write React components, allowing us to manage state and side effects in a more intuitive and maintainable way. By adopting hooks, you can create cleaner, more modular code, leading to better overall application architecture.