Mastering State Management with Redux Toolkit
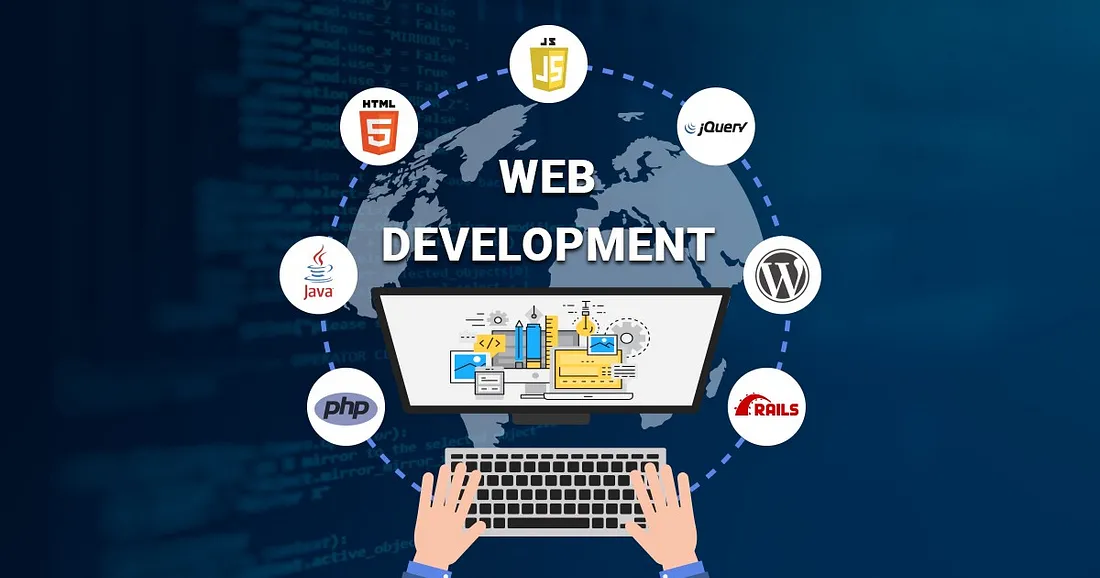
Introduction
State management is a critical aspect of building React applications, especially as they grow in complexity. Redux has been a popular choice for managing global state, but it often comes with a lot of boilerplate code. Redux Toolkit aims to address this by providing a more concise and opinionated way to write Redux logic.
What is Redux Toolkit?
Redux Toolkit is the official, recommended way to write Redux logic. It includes a set of tools and best practices that make it easier to manage global state. With features like createSlice
, createAsyncThunk
, and the configureStore
API, Redux Toolkit reduces boilerplate and encourages better code organization.
Creating a Slice
A slice in Redux Toolkit represents a portion of your Redux state and the actions that can modify that state. Here's an example of creating a counter slice:
import { createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: 0,
reducers: {
increment: state => state + 1,
decrement: state => state - 1,
},
});
export const { increment, decrement } = counterSlice.actions;
export default counterSlice.reducer;
Async Logic with createAsyncThunk
Redux Toolkit also simplifies handling asynchronous logic. The createAsyncThunk
function allows you to define async actions in a straightforward manner:
import { createAsyncThunk } from '@reduxjs/toolkit';
import axios from 'axios';
export const fetchUser = createAsyncThunk(
'user/fetchUser',
async (userId: string) => {
const response = await axios.get(`/api/user/${userId}`);
return response.data;
}
);
Benefits of Redux Toolkit
- Reduced Boilerplate: The concise APIs provided by Redux Toolkit eliminate the need for repetitive code.
- Better Code Organization: By grouping actions and reducers in slices, your Redux code becomes more modular and easier to maintain.
- Built-in Best Practices: Redux Toolkit encourages the use of best practices, such as immutability and avoiding side effects in reducers.
Conclusion
Redux Toolkit is a powerful tool that simplifies state management in React applications. By adopting Redux Toolkit, you can write cleaner, more maintainable code while benefiting from the performance and organizational improvements it offers.